Using String.join Method in Java: Your Ultimate Guide
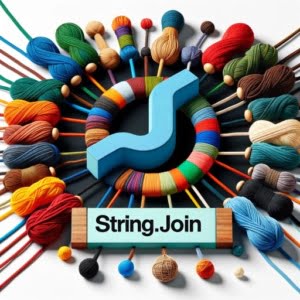
Are you finding it challenging to join strings in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling string concatenation in Java, but we’re here to help.
Think of the join()
method in Java as a bridge, connecting individual strings into one cohesive unit. This method is a powerful tool in Java, providing a versatile and handy solution for various string manipulation tasks.
In this guide, we’ll walk you through the join()
method in Java, from its basic usage to more advanced techniques. We’ll cover everything from the basics of string concatenation to more complex scenarios, as well as alternative approaches.
Let’s get started and master string joining in Java!
TL;DR: How Do I Join Strings in Java?
In Java, you can join strings using the
String.join()
method followed by a comma separated list of strings, ie:String newString = String.join("New", "-", "String");
. This method allows you to concatenate multiple strings with a specified delimiter.
Here’s a simple example:
String result = String.join("-", "Hello", "World");
System.out.println(result);
// Output:
// 'Hello-World'
In this example, we use the String.join()
method to join two strings, ‘Hello’ and ‘World’, with a hyphen (‘-‘) as the delimiter. The result is a single string ‘Hello-World’.
This is just a basic way to use the
String.join()
method in Java. But there’s much more to learn about string concatenation in Java. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Basic Usage of String.join in Java
- Advanced String Joining: Arrays and Collections
- Exploring Alternatives to String.join in Java
- Troubleshooting String.join in Java
- Understanding Java’s String Class
- Expanding Your Knowledge: Real-World Applications and Further Concepts
- Further Resources for Mastering String Manipulation in Java
- Wrapping Up: Mastering String.join in Java
Basic Usage of String.join in Java
The String.join()
method in Java is a simple and effective way to concatenate, or join, strings. This method takes in a delimiter and an array of strings, and returns a single string that has all the strings joined with the specified delimiter.
The syntax for using the String.join()
method is as follows:
String.join(delimiter, elements);
Here, delimiter
is the character or string that separates each element, and elements
are the strings to be joined.
Let’s look at a basic example:
String str1 = "Hello";
String str2 = "World";
String result = String.join("-", str1, str2);
System.out.println(result);
// Output:
// 'Hello-World'
In this example, we have two strings, ‘Hello’ and ‘World’. We use the String.join()
method with a hyphen (‘-‘) as the delimiter to join these two strings. The result is ‘Hello-World’.
The String.join()
method is a powerful tool for string concatenation in Java. It’s simple to use, and it allows you to join any number of strings with a specified delimiter. However, it’s important to note that the String.join()
method doesn’t handle null values well. If any of the elements are null, the method will throw a NullPointerException. Therefore, it’s always a good practice to check for null values before using the String.join()
method.
Advanced String Joining: Arrays and Collections
As you delve deeper into Java, you’ll often find yourself dealing with arrays of strings or collections. The String.join()
method shows its true power in these scenarios, allowing you to join an entire array or collection of strings with a single line of code.
Joining Arrays of Strings
Let’s start with an example of joining an array of strings:
String[] words = {"Java", "is", "awesome"};
String sentence = String.join(" ", words);
System.out.println(sentence);
// Output:
// 'Java is awesome'
In this example, we have an array of strings words
. We use the String.join()
method with a space (‘ ‘) as the delimiter to join all the strings in the array. The result is a single string ‘Java is awesome’.
Joining Collections of Strings
The String.join()
method can also be used with collections of strings. Here’s how you can do it:
List<String> wordsList = Arrays.asList("Java", "is", "powerful");
String sentenceList = String.join(" ", wordsList);
System.out.println(sentenceList);
// Output:
// 'Java is powerful'
This time, we have a List of strings wordsList
. Again, we use the String.join()
method with a space (‘ ‘) as the delimiter to join all the strings in the List. The result is a single string ‘Java is powerful’.
These examples illustrate the versatility of the String.join()
method in Java. Whether you’re working with arrays or collections of strings, String.join()
provides an easy and efficient way to concatenate strings. However, remember to handle null values appropriately to prevent any NullPointerExceptions.
Exploring Alternatives to String.join in Java
While String.join()
is a powerful tool for string concatenation in Java, it’s not the only one. There are several other methods you can use to join strings, such as using the +
operator, StringBuilder
, StringBuffer
, or String.format()
. Each of these methods has its own advantages and disadvantages. Let’s explore each of these alternatives.
Using the +
Operator
The +
operator is the simplest way to join strings in Java. Here’s an example:
String str1 = "Hello";
String str2 = "World";
String result = str1 + "-" + str2;
System.out.println(result);
// Output:
// 'Hello-World'
While the +
operator is simple and easy to use, it’s not the most efficient method for joining strings, especially when dealing with a large number of strings.
Using StringBuilder
StringBuilder
is a class in Java that’s used for creating mutable strings. It’s particularly useful when you need to concatenate strings in a loop.
StringBuilder sb = new StringBuilder();
sb.append("Hello");
sb.append("-");
sb.append("World");
System.out.println(sb.toString());
// Output:
// 'Hello-World'
StringBuilder
is more efficient than the +
operator when concatenating multiple strings. However, it’s not as straightforward to use.
Using StringBuffer
StringBuffer
is similar to StringBuilder
, but it’s thread-safe. This means that it can be used safely in multithreaded environments.
StringBuffer sbf = new StringBuffer();
sbf.append("Hello");
sbf.append("-");
sbf.append("World");
System.out.println(sbf.toString());
// Output:
// 'Hello-World'
While StringBuffer
is thread-safe, it’s less efficient than StringBuilder
due to the overhead of synchronization.
Using String.format()
String.format()
is another method for joining strings in Java. It allows you to create formatted strings using placeholders.
String result = String.format("%s-%s", "Hello", "World");
System.out.println(result);
// Output:
// 'Hello-World'
String.format()
provides a lot of flexibility for creating formatted strings, but it can be overkill for simple string concatenation tasks.
Here’s a comparison table to help you choose the most suitable method for your needs:
Method | Advantages | Disadvantages |
---|---|---|
+ Operator | Simple and easy to use | Not efficient for joining multiple strings |
StringBuilder | Efficient for multiple strings | Not as straightforward to use |
StringBuffer | Thread-safe | Less efficient due to synchronization |
String.format() | Flexible for formatted strings | Overkill for simple tasks |
While String.join()
is a versatile and efficient method for joining strings in Java, understanding these alternative methods will give you more tools to handle string concatenation in different scenarios.
Troubleshooting String.join in Java
While String.join()
is a powerful method for joining strings in Java, it’s not without its potential issues. Here, we’ll discuss some of the common problems you might encounter when using String.join()
, along with solutions and workarounds.
Handling Null Pointer Exceptions
One common issue is the Null Pointer Exception. This occurs when one of the elements you’re trying to join is null. Here’s an example:
String str1 = null;
String str2 = "World";
// This will throw a NullPointerException
String result = String.join("-", str1, str2);
To avoid this, you should always check for null values before using String.join()
:
String str1 = null;
String str2 = "World";
// Check for null values
if (str1 != null && str2 != null) {
String result = String.join("-", str1, str2);
System.out.println(result);
} else {
System.out.println("One or more values are null");
}
Performance Considerations
When joining a large number of strings, performance can become a concern. In such cases, using StringBuilder
or StringBuffer
might be more efficient than String.join()
. However, String.join()
is usually more than adequate for most use cases.
Other Considerations
Remember that String.join()
uses a specified delimiter to join strings. If you don’t want any delimiter, you can pass an empty string as the delimiter.
String str1 = "Hello";
String str2 = "World";
String result = String.join("", str1, str2);
System.out.println(result);
// Output:
// 'HelloWorld'
In this example, we’re joining two strings without any delimiter. The result is a single string ‘HelloWorld’.
Understanding these potential issues and how to handle them will help you use String.join()
effectively and efficiently in your Java code.
Understanding Java’s String Class
Before we delve deeper into string concatenation methods like String.join()
, it’s important to understand the fundamentals of Java’s String class.
In Java, string is an object that represents a sequence of characters. The java.lang.String
class is used to create and manipulate strings.
String str = "Hello, World!";
System.out.println(str);
// Output:
// 'Hello, World!'
In this example, we create a string using the String
class and print it to the console. The output is ‘Hello, World!’.
Immutability of Strings in Java
One key characteristic of strings in Java is their immutability. Once a string object is created, it cannot be changed. This might seem counterintuitive when we talk about string manipulation, but what actually happens is that a new string object is created whenever a change is made.
String str = "Hello";
str = str + ", World!";
System.out.println(str);
// Output:
// 'Hello, World!'
In this example, when we append ‘, World!’ to the string ‘Hello’, a new string object ‘Hello, World!’ is created. The original string ‘Hello’ remains unchanged.
This immutability of strings has several implications for string manipulation in Java. For example, when concatenating strings using the +
operator in a loop, a new string object is created at each iteration, which can lead to performance issues. Methods like String.join()
, StringBuilder
, and StringBuffer
are designed to address these issues, providing more efficient ways to manipulate strings.
Understanding these fundamentals will give you a solid foundation to fully grasp and effectively use string concatenation methods like String.join()
in Java.
Expanding Your Knowledge: Real-World Applications and Further Concepts
Understanding String.join()
and other string concatenation methods in Java is just the beginning. The ability to manipulate strings effectively can open up a world of possibilities in many different applications.
String Joining in File Handling
In file handling, for example, you might need to construct file paths or read and write data from and to files. The String.join()
method can be extremely useful in these scenarios, allowing you to concatenate strings effectively and efficiently.
String Joining in Data Processing
In data processing, you often need to manipulate and transform data. This often involves joining strings to create identifiers, labels, or other types of data. Again, String.join()
and other string concatenation methods can be invaluable tools.
Exploring Related Concepts
Once you’ve mastered string joining in Java, you might want to explore related concepts like string formatting and regular expressions. String formatting allows you to create complex, formatted strings, while regular expressions provide a powerful tool for pattern matching and text manipulation.
Further Resources for Mastering String Manipulation in Java
To deepen your understanding of string manipulation in Java, consider checking out the following resources:
- Advanced Techniques for Using the String Class in Java – Discover Java String internationalization support.
Using ReplaceAll in Java – Explore regex patterns and replacement strings for versatile string manipulation.
Java Substring Functionality – Master substring extraction techniques for string manipulation in Java
Oracle’s Java Documentation – The official documentation for the String class in Java.
Java String Class Tutorial by DigitalOcean covers various methods for string manipulation.
Java String Handling Tutorial by JavaTpoint is another excellent tutorial on string handling in Java.
Remember, mastering a programming language is a journey. As you continue to learn and practice, you’ll discover new tools and techniques, and become a more skilled and efficient programmer.
Wrapping Up: Mastering String.join in Java
In this comprehensive guide, we’ve unraveled the intricacies of using String.join()
in Java, a key method for string concatenation.
We began with the basics, understanding how to use String.join()
to merge multiple strings using a specified delimiter. We then delved into more advanced usage, discussing how to use String.join()
with arrays and collections, and how to handle potential issues such as null pointer exceptions.
We also explored alternative methods for joining strings, such as the +
operator, StringBuilder
, StringBuffer
, and String.format()
. Each of these methods has its unique advantages and trade-offs, giving you a broader toolkit for handling string concatenation in Java.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantages | Disadvantages |
---|---|---|
String.join() | Versatile, easy to use | Doesn’t handle null values well |
+ Operator | Simple and easy to use | Not efficient for joining multiple strings |
StringBuilder | Efficient for multiple strings | More complex to use |
StringBuffer | Thread-safe | Less efficient due to synchronization |
String.format() | Flexible for formatted strings | Overkill for simple tasks |
Whether you’re just starting out with String.join()
or you’re looking to deepen your understanding of string concatenation in Java, we hope this guide has been a valuable resource.
With the knowledge and skills you’ve gained, you’re now well-equipped to handle any string concatenation task in Java. Happy coding!