Python Increment By 1 | Quick and Easy Examples
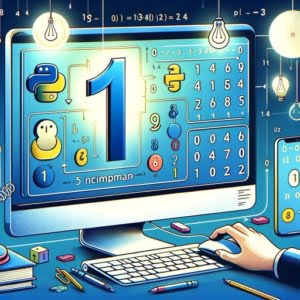
Have you ever been puzzled about why Python, unlike languages such as C++ or JavaScript, doesn’t include increment and decrement operators? You’re not alone. This seemingly simple feature, found in many other languages, is noticeably missing in Python.
But don’t worry — you can work around this. In this blog post, we’re going to explore the reasons behind Python’s unique approach and provide you with Pythonic alternatives to achieve similar functionality.
By the end of this post, you’ll have a clear understanding of how to manipulate values in Python, including incrementing and decrementing numerical values and strings. So, if you’re ready to take this Python journey, let’s start climbing!
TL;DR: How do you increment by 1 in Python?
To increment a variable
x
by 1 in Python, you can use the addition assignment operator+=
. The expressionx += 1
means increase the value ofx
by 1.
Example:
x = 5
x += 1
print(x) # outputs: 6
Table of Contents
The Importance of Increment and Decrement Operators
Before we explore Python’s unique approach, it’s essential to understand what increment and decrement operators are.
Language | Increment | Decrement |
---|---|---|
C++ | ++ | -- |
JavaScript | ++ | -- |
Python | += | -= |
In programming, increment operators are used to increase the value of a variable by a certain amount, typically by 1. On the other hand, decrement operators decrease the value of a variable by a certain amount, usually by 1. Languages like C++ and JavaScript accomplish this using the ‘++’ and ‘–‘ operators respectively.
The Python Way
If you’ve worked with Python, you’ll know that it doesn’t include these operators. Instead, Python employs different methods to increment and decrement values, which we’ll explore in the subsequent sections.
Python’s approach ties closely to the concept of immutability.
Example of immutability in Python:
x = 5
print(id(x)) # prints the id of x
x += 1
print(id(x)) # prints the id of x after incrementing
Certain data types in Python, like integers and strings, are immutable. This means that once a value is assigned to a variable of these types, the value can’t be altered without creating a new object.
At first glance, this may seem like an odd approach. But it’s a fundamental aspect of Python’s philosophy. Python emphasizes code readability and simplicity, often referred to as the ‘Pythonic way’.
So, while Python might lack certain features found in other languages, like increment and decrement operators, it compensates with its simplicity and readability. The absence of ‘++’ and ‘–‘ operators might initially seem like a disadvantage, but it’s a deliberate design choice that adds to Python’s unique charm.
Python Equivalents to Increment and Decrement Operators
Now that we’ve understood why Python does not include increment and decrement operators, let’s look at how we can simulate these operations in Python. To do this, we employ two operators you might already be familiar with: +=
and -=
.
In Python, the +=
operator is used to increment a value. For instance, if you have a variable x
with a value of 5, you can increment it by 1 using x += 1
.
After this operation, x
will hold a value of 6. Similarly, the -=
operator is used to decrement a value. If we take our variable x
with a value of 6, we can decrement it by 1 using x -= 1
. After this operation, x
will again hold a value of 5.
x = 5
x += 1 # x is now 6
x -= 1 # x is now 5
Mutability and Incrementing
Consider the id()
function in Python, which returns the identity of an object. This identity is unique and constant for the object during its lifetime. If we print the id of x
before and after incrementing it, you’ll see that the ids are different.
x = 5
print(id(x)) # prints the id of x
x += 1
print(id(x)) # prints the id of x after incrementing
This demonstrates that when we incremented x
, we didn’t modify the original value. Instead, we created a new value (6) and assigned it to x
.
When you increment or decrement a value in Python, you’re not modifying the original value. Instead, you’re creating a new value and assigning it to the variable. This is because integers are immutable in Python.
Advanced Usage
The +=
operator in Python is quite flexible. It can increment a value by any number, not just 1. For example, x += 3
will increment x
by 3.
Additionally, Python’s for
loop can increment by custom values using the step
parameter within the range()
function. For example, the following code will print the numbers 0 to 9, incrementing by 2 each time.
for i in range(0, 10, 2):
print(i)
This flexibility and the ability to clearly express your intent is part of what makes Python a powerful and popular language.
Decrementing Values
Just as we can increment values in Python using the +=
operator, we can also decrement values using the -=
operator. For instance, if we have a variable x
with a value of 5, we can decrement it by 1 using x -= 1
. This will result in x
having a value of 4.
x = 5
x -= 1 # x is now 4
While Loops and Incremention
Let’s delve into another crucial concept in Python: while loops. A while loop in Python executes a target statement repeatedly as long as a given condition holds true.
Here’s how you can use a while loop to increment a value:
x = 0
while x < 5:
x += 1
print(x)
This code will print the numbers 1 to 5. It starts with x
at 0 and increments x
by 1 in each iteration of the loop, halting once x
is no longer less than 5.
You can also embed conditions within loops for more complex operations. For example, you could increment a value within a while loop, but only if the value meets a certain condition.
x = 0
while x < 10:
x += 1
if x % 2 == 0:
print(x)
This code will increment x
by 1 in each iteration of the loop, but it will only print x
if x
is an even number.
“Incrementing” Strings in Python
Up until now, we’ve discussed how to increment and decrement numerical values in Python. But what about strings? Is it possible to increment them as well?
In Python, strings, like integers, are immutable sequences of characters. This means that once a string is created, it cannot be changed. Any operation that appears to modify a string will actually create a new string.
Despite this immutability, Python offers a powerful way to ‘increment’ strings, or to be more precise, to concatenate strings, using the same +=
operator used with integers.
For example, if we have a string str1
with a value of ‘Hello’, we can ‘increment’ it by another string using str1 += ', World!'
. This will result in str1
having a value of ‘Hello, World!’.
str1 = 'Hello'
str1 += ', World!'
# str1 is now 'Hello, World!'
Although this is string concatenation, you can think of it as incrementing a string with the “increment quantity” being the contents second string! This is because the syntax is the same as incrementing an integer by a value other than the default of 1.
It’s important to note that the
+=
operator can only be used to concatenate strings with other strings. If you try to concatenate a string with a non-string type, such as an integer, Python will throw a TypeError.
Example of TypeError when trying to concatenate a string with an integer:
str1 = 'Hello'
str1 += 5 # Raises TypeError: can only concatenate str (not "int") to str
Type Conversion for String Concatenation
This is where type conversion comes into play. In Python, you can convert one data type to another using built-in functions like str()
, int()
, and float()
. So if you need to concatenate a string with a number, you can convert the number to a string first.
str1 = 'Hello'
str1 += str(5) # str1 is now 'Hello5'
Further Resources for Math Operators
Check out these extra resources to learn more about Python Math and Operators:
- Mastering Math Operations in Python – Learn how to perform common mathematical calculations using Python’s built-in math functions.
Exploring NaN in Python – Learn how NaN is used in Python for numerical computations and handling missing data.
Exploring NaN in Python – Learn how NaN is used in Python for numerical computations and handling missing data.
Understanding the Double Slash Operator in Python – A quick guide to deciphering the use of the double slash operator in Python.
Official Python Library for Operator Module – Access the full documentation for Python’s operator module.
How to Increment Numbers in Python – Discover effective strategies to increment numbers in your Python code.
Understanding Python Math Operators can make your job easier when you’re dealing with math or math related problems in programming.
Wrapping Up
In this article, we’ve discovered that Python’s non traditional increment operators aren’t meant as a limitation, but rather a design choice that aligns with Python’s philosophy of simplicity and readability.
We’ve explored how Python uses the +=
and -=
operators to emulate increment and decrement operations. We’ve seen how these operators, combined with Python’s powerful looping constructs and condition statements, provide a flexible and expressive way to manipulate data.
We’ve also delved into the concept of immutability in Python, understanding that operations like incrementing and decrementing don’t modify the original data, but instead create new data.
Finally, we’ve seen how Python allows us to ‘increment’ strings through concatenation, and how type conversion plays a crucial role when working with different data types.
If you found this tutorial insightful, don’t miss our detailed cheat sheet on Python syntax.
Understanding these concepts is key to writing effective Python code. So while Python might not have ++
or --
operators, it offers an equally powerful, if not more expressive, way to manipulate your data. And that’s the beauty of Python.